- Why Temu's bargain prices are about to hit a tariff wall
- Surge in threat actors scanning Juniper, Cisco, and Palo Alto Networks devices
- Ivanti warns customers of new critical flaw exploited in the wild
- Why this Bluetooth transmitter is a must-have for frequent travelers - especially at this price
- Kindle's new AI recap feature helps bring you up to speed - how to try it
Master Docker and VS Code: Supercharge Your Dev Workflow | Docker
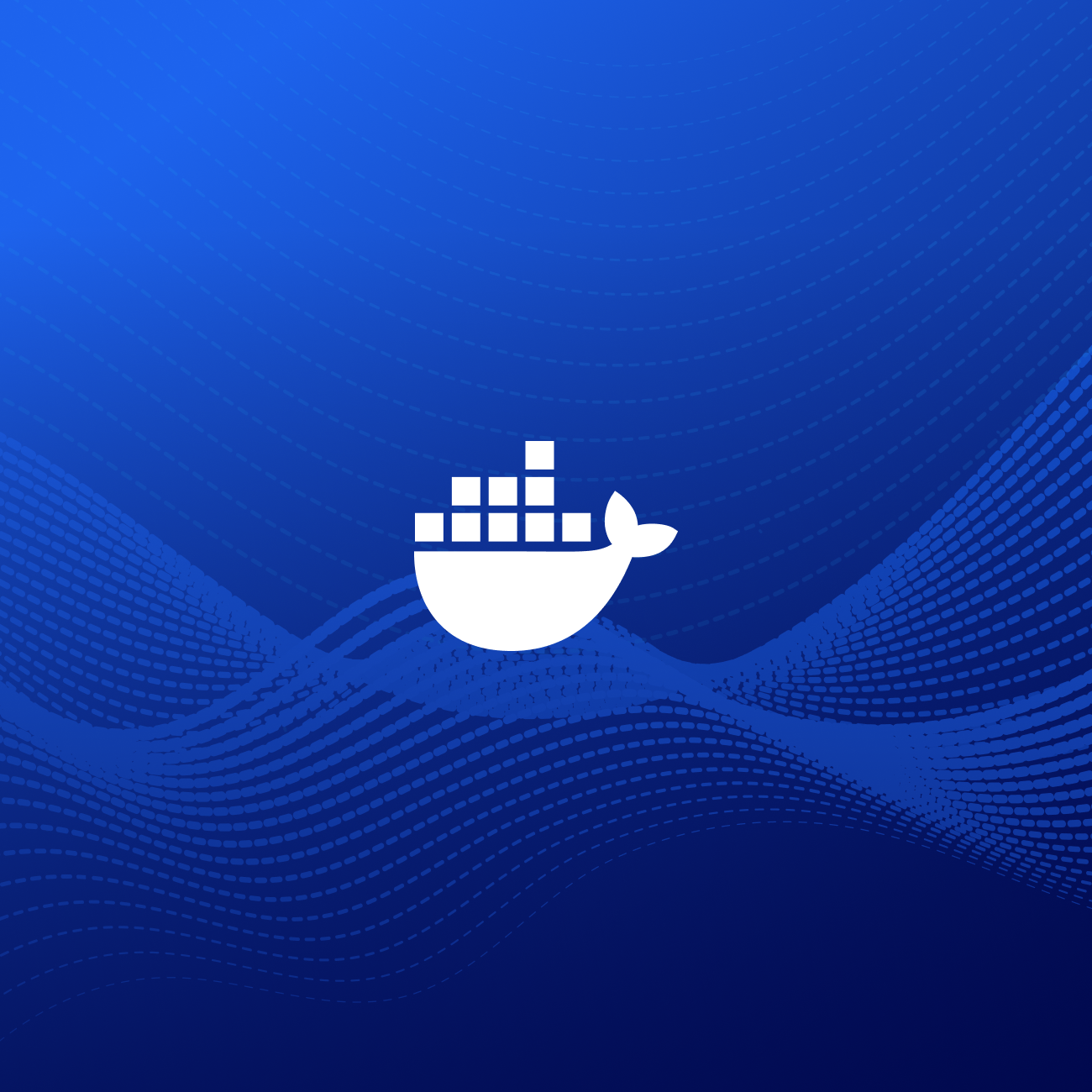
Hey there, fellow developers and DevOps champions! I’m excited to share a workflow that has transformed my teams’ development experience time and time again: pairing Docker and Visual Studio Code (VS Code). If you’re looking to speed up local development, minimize those “it works on my machine” excuses, and streamline your entire workflow, you’re in the right place.
I’ve personally encountered countless environment mismatches during my career, and every time, Docker plus VS Code saved the day — no more wasted hours debugging bizarre OS/library issues on a teammate’s machine.
In this post, I’ll walk you through how to get Docker and VS Code humming in perfect harmony, cover advanced debugging scenarios, and even drop some insider tips on security, performance, and ephemeral development environments. By the end, you’ll be equipped to tackle DevOps challenges confidently.
Let’s jump in!
Why use Docker with VS Code?
Whether you’re working solo or collaborating across teams, Docker and Visual Studio Code work together to ensure your development workflow remains smooth, scalable, and future-proof. Let’s take a look at some of the most notable benefits to using Docker with VS Code.
- Consistency Across Environments
Docker’s containers standardize everything from OS libraries to dependencies, creating a consistent dev environment. No more “it works on my machine!” fiascos. - Faster Development Feedback Loop
VS Code’s integrated terminal, built-in debugging, and Docker extension cut down on context-switching—making you more productive in real time. - Multi-Language, Multi-Framework
Whether you love Node.js, Python, .NET, or Ruby, Docker packages your app identically. Meanwhile, VS Code’s extension ecosystem handles language-specific linting and debugging. - Future-Proof
By 2025, ephemeral development environments, container-native CI/CD, and container security scanning (e.g., Docker Scout) will be table stakes for modern teams. Combining Docker and VS Code puts you on the fast track.
Together, Docker and VS Code create a streamlined, flexible, and reliable development environment.
How Docker transformed my development workflow
Let me tell you a quick anecdote:
A few years back, my team spent two days diagnosing a weird bug that only surfaced on one developer’s machine. It turned out they had an outdated system library that caused subtle conflicts. We wasted hours! In the very next sprint, we containerized everything using Docker. Suddenly, everyone’s environment was the same — no more “mismatch” drama. That was the day I became a Docker convert for life.
Since then, I’ve recommended Dockerized workflows to every team I’ve worked with, and thanks to VS Code, spinning up, managing, and debugging containers has become even more seamless.
How to set up Docker in Visual Studio Code
Setting up Docker in Visual Studio Code can be done in a matter of minutes. In two steps, you should be well on your way to leveraging the power of this dynamic duo. You can use the command line to manage containers and build images directly within VS Code. Also, you can create a new container with specific Docker run parameters to maintain configuration and settings.
But first, let’s make sure you’re using the right version!
Ensuring version compatibility
As of early 2025, here’s what I recommend to minimize friction:
- Docker Desktop: v4.37+ or newer (Windows, macOS, or Linux)
- Docker Engine: 27.5+ (if running Docker directly on Linux servers)
- Visual Studio Code: 1.96+
Lastly, check the Docker release notes and VS Code updates to stay aligned with the latest features and patches. Trust me, staying current prevents a lot of “uh-oh” moments later.
1. Docker Desktop (or Docker Engine on Linux)
2. Visual Studio Code
- Install from VS Code’s website.
- Docker Extension: Press
Ctrl+Shift+X
(Windows/Linux) orCmd+Shift+X
(macOS) in VS Code → search for “Docker” → Install. - Explore: You’ll see a whale icon on the left toolbar, giving you a GUI-like interface for Docker containers, images, and registries.
Pro Tip: If you’re going fully DevOps, keep Docker Desktop and VS Code up to date. Each release often adds new features or performance/security enhancements.
Examples: Building an app with a Docker container
Node.js example
We’ll create a basic Node.js web server using Express, then build and run it in Docker. The server listens on port 3000 and returns a simple greeting.
Create a Project Folder:
mkdir node-docker-app
cd node-docker-app
Initialize a Node.js Application:
npm init -y
npm install express
- ‘npm init -y’ generates a default package.json.
- ‘npm install express’ pulls in the Express framework and saves it to ‘package.json’.
Create the Main App File (index.js):
cat <index.js
const express = require('express');
const app = express();
const port = 3000;
app.get('/', (req, res) => {
res.send('Hello from Node + Docker + VS Code!');
});
app.listen(port, () => {
console.log(`App running on port ${port}`);
});
EOF
- This code starts a server on port 3000. When a user hits http://localhost:3000, they see a “Hello from Node + Docker + VS Code!” message.
Add an Annotated Dockerfile:
# Use a lightweight Node.js 23.x image based on Alpine Linux
FROM node:23.6.1-alpine
# Set the working directory inside the container
WORKDIR /usr/src/app
# Copy only the package files first (for efficient layer caching)
COPY package*.json ./
# Install Node.js dependencies
RUN npm install
# Copy the entire project (including index.js) into the container
COPY . .
# Expose port 3000 for the Node.js server (metadata)
EXPOSE 3000
# The default command to run your app
CMD ["node", "index.js"]
Build and run the container image:
docker build -t node-docker-app .
Run the container, mapping port 3000 on the container to port 3000 locally:
docker run -p 3000:3000 node-docker-app
Python example
Here, we’ll build a simple Flask application that listens on port 3001 and displays a greeting. We’ll then package it into a Docker container.
Create a Project Folder:
mkdir python-docker-app
cd python-docker-app
Create a Basic Flask App (app.py):
cat <app.py
from flask import Flask
app = Flask(__name__)
@app.route('/')
def hello():
return 'Hello from Python + Docker!'
if __name__ == '__main__':
app.run(host='0.0.0.0', port=3001)
EOF
- This code defines a minimal Flask server that responds to requests on port 3001.
Add Dependencies:
echo "Flask==2.3.0" > requirements.txt
- Lists your Python dependencies. In this case, just Flask.
Create an Annotated Dockerfile:
# Use Python 3.11 on Alpine for a smaller base image
FROM python:3.11-alpine
# Set the container's working directory
WORKDIR /app
# Copy only the requirements file first to leverage caching
COPY requirements.txt .
# Install Python libraries
RUN pip install -r requirements.txt
# Copy the rest of the application code
COPY . .
# Expose port 3001, where Flask will listen
EXPOSE 3001
# Run the Flask app
CMD ["python", "app.py"]
Build and Run:
docker build -t python-docker-app .
docker run -p 3001:3001 python-docker-app
- Visit http://localhost:3001 and you’ll see “Hello from Python + Docker!”
Manage containers in VS Code with the Docker Extension
Once you install the Docker extension in Visual Studio Code, you can easily manage containers, images, and registries — bringing the full power of Docker into VS Code. You can also open a code terminal within VS Code to execute commands and manage applications seamlessly through the container’s isolated filesystem. And the context menu can be used to perform actions like starting, stopping, and removing containers.
With the Docker extension installed, open VS Code and click the Docker icon:
- Containers: View running/stopped containers, view logs, stop, or remove them at a click. Each container is associated with a specific container name, which helps you identify and manage them effectively.
- Images: Inspect your local images, tag them, or push to Docker Hub/other registries.
- Registries: Quickly pull from Docker Hub or private repos.
No more memorizing container IDs or retyping long CLI commands. This is especially handy for visual folks or those new to Docker.
Advanced Debugging (Single & Multi-Service)
Debugging containerized applications can be challenging, but with Docker and Visual Studio Code, you can streamline the process. Here’s some advanced debugging that you can try for yourself!
- Containerized Debug Ports
- For Node, expose port
9229
(EXPOSE 9229
) and run with--inspect
. - In VS Code, create a “Docker: Attach to Node” debug config to step through code in real time.
- For Node, expose port
- Microservices / Docker Compose
- For multiple services, define them in
compose.yml
. - Spin them up with
docker compose up
. - Configure VS Code to attach debuggers to each service’s port (e.g., microservice A on
9229
, microservice B on9230
).
- For multiple services, define them in
- Remote – Containers (Dev Containers)
- Use VS Code’s Dev Containers extension for a fully containerized development environment.
- Perfect for large teams: Everyone codes in the same environment with preinstalled tools/libraries. Onboarding new devs is a breeze.
Insider Trick: If you’re juggling multiple services, label your containers meaningfully (--name web-service
, --name auth-service
) so they’re easy to spot in VS Code’s Docker panel.
Pro Tips: Security & Performance
Security
- Use Trusted Base Images
Official images likenode:23.6.1-alpine, python:3.11-alpine
, etc., reduce the risk of hidden vulnerabilities. - Scan Images
Tools like Docker Scout or Trivy spot vulnerabilities. Integrate into CI/CD to catch them early. - Secrets Management
Never hardcode tokens or credentials—use Docker secrets, environment variables, or external vaults. - Encryption & SSL
For production apps, consider a reverse proxy (Nginx, Traefik) or in-container SSL termination.
Performance
- Resource Allocation
Docker Desktop on Windows/macOS allows you to tweak CPU/RAM usage. Don’t starve your containers if you run multiple services. - Multi-Stage Builds &
.dockerignore
Keep images lean and build times short by ignoring unneeded files (node_modules
,.git
). - Dev Containers
Offload heavy dependencies to a container, freeing your host machine. - Docker Compose v2
It’s the new standard—faster, more intuitive commands. Great for orchestrating multi-service setups on your dev box.
Real-World Impact: In a recent project, containerizing builds and using ephemeral agents slashed our development environment setup time by 80%. That’s more time coding, and less time wrangling dependencies!
Going Further: CI/CD and Ephemeral Dev Environments
CI/CD Integration:
Automate Docker builds in Jenkins, GitHub Actions, or Azure DevOps. Run your tests within containers for consistent results.
# Example GitHub Actions snippet
jobs:
build-and-test:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v4
- name: Build Docker Image
run: docker build -t myorg/myapp:${{ github.sha }} .
- name: Run Tests
run: docker run --rm myorg/myapp:${{ github.sha }} npm test
- name: Push Image
run: |
docker login -u $USER -p $TOKEN
docker push myorg/myapp:${{ github.sha }}
- Ephemeral Build Agents:
Use Docker containers as throwaway build agents. Each CI job spins up a clean environment—no leftover caches or config drift. - Docker in Kubernetes:
If you need to scale horizontally, deploying containers to Kubernetes (K8s) can handle higher traffic or more complex microservice architectures. - Dev Containers & Codespaces:
Tools like GitHub Codespaces or the “Remote – Containers” extension let you develop in ephemeral containers in the cloud. Perfect for distributed teams—everyone codes in the same environment, minus the “It’s fine on my laptop!” tension.
Conclusion
Congrats! You’ve just stepped through how Docker and Visual Studio Code can supercharge your development:
- Consistent Environments: Docker ensures no more environment mismatch nightmares.
- Speedy Debugging & Management: VS Code’s integrated Docker extension and robust debug tools keep you in the zone.
- Security & Performance: Multi-stage builds, scans, ephemeral dev containers—these are the building blocks of a healthy, future-proofed DevOps pipeline.
- CI/CD & Beyond: Extend the same principles to your CI/CD flows, ensuring smooth releases and easy rollbacks.
Once you see the difference in speed, reliability, and consistency, you’ll never want to go back to old-school local setups.
And that’s it! We’ve packed in real-world anecdotes, pro tips, stories, and future-proof best practices to impress seasoned IT pros on Docker.com. Now go forth, embrace containers, and code with confidence — happy shipping!
Learn more
- Install or upgrade Docker Desktop & VS Code to the latest versions.
- Try containerizing an app from scratch — your future self (and your teammates) will thank you.
- Experiment with ephemeral dev containers, advanced debugging, or Docker security scanning (Docker Scout).
- Join the Docker community on Slack or the forums. Swap stories or pick up fresh tips!